Collapsing numbers
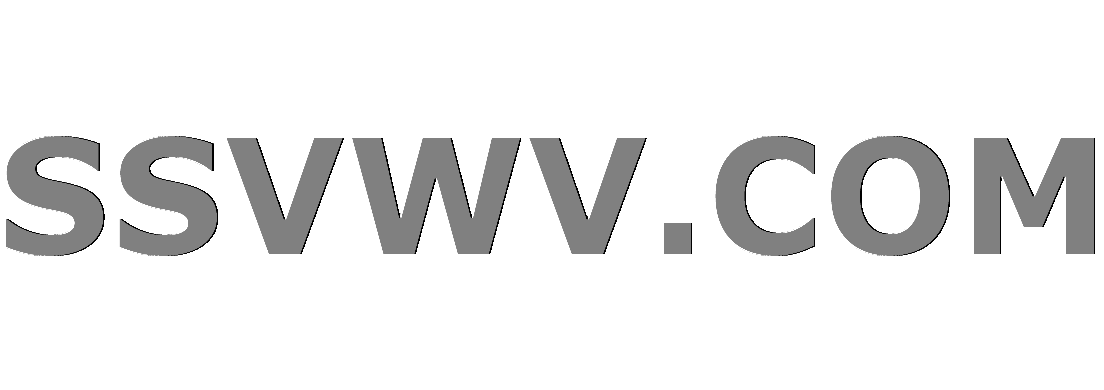
Multi tool use
Let's define the a function on natural numbers $n$, written as base 10 digits $d_k; d_{k-1}; dotsc; d_1; d_0$, as follows:
As long as there are equal adjacent digits $d_i;d_{i-1}$, replace them by their sum $d_i+d_{i-1}$ from left to right. If there were any such digits, repeat the same procedure.
In other words, in each iteration we greedily take all pairs of equal adjacent digits and replace them by their sum at the same time (using the left-most pair if they overlap).
Example
Let's take $texttt{9988}$ for example:
- The first adjacent digits which are equal are the two $texttt{9}$
- So we replace them by $texttt{9 + 9} = texttt{18}$ which gives us $texttt{1888}$
- Since we're still in the first left-right traversal and there were still two $texttt{8}$s we need to first replace these
- So we get $texttt{1816}$
- Something changed, so we need to do another iteration
- But there are no such digits, so we stop
Therefore the $9988^text{th}$ number in that sequence is $1816$.
Challenge
The first 200 terms are:
0,1,2,3,4,5,6,7,8,9,10,2,12,13,14,15,16,17,18,19,20,21,4,23,24,25,26,27,28,29,30,31,32,6,34,35,36,37,38,39,40,41,42,43,8,45,46,47,48,49,50,51,52,53,54,10,56,57,58,59,60,61,62,63,64,65,12,67,68,69,70,71,72,73,74,75,76,14,78,79,80,81,82,83,84,85,86,87,16,89,90,91,92,93,94,95,96,97,98,18,10,101,102,103,104,105,106,107,108,109,20,21,4,23,24,25,26,27,28,29,120,121,14,123,124,125,126,127,128,129,130,131,132,16,134,135,136,137,138,139,140,141,142,143,18,145,146,147,148,149,150,151,152,153,154,20,156,157,158,159,160,161,162,163,164,165,4,167,168,169,170,171,172,173,174,175,176,24,178,179,180,181,182,183,184,185,186,187,26,189,190,191,192,193,194,195,196,197,198,28
Your task is to generate that sequence, either
- given $n$, return the $n^text{th}$ number in that sequence,
- given $n$, return the first $n$ numbers in that sequence
- or generate the sequence indefinitely.
You may choose your submission to use either $0$- or $1$-indexing, but please specify which.
Test cases
You may use the above given terms, however here are some larger ones:
222 -> 42
1633 -> 4
4488 -> 816
15519 -> 2019
19988 -> 2816
99999 -> 18189
119988 -> 21816
100001 -> 101
999999 -> 181818
code-golf sequence integer
add a comment |
Let's define the a function on natural numbers $n$, written as base 10 digits $d_k; d_{k-1}; dotsc; d_1; d_0$, as follows:
As long as there are equal adjacent digits $d_i;d_{i-1}$, replace them by their sum $d_i+d_{i-1}$ from left to right. If there were any such digits, repeat the same procedure.
In other words, in each iteration we greedily take all pairs of equal adjacent digits and replace them by their sum at the same time (using the left-most pair if they overlap).
Example
Let's take $texttt{9988}$ for example:
- The first adjacent digits which are equal are the two $texttt{9}$
- So we replace them by $texttt{9 + 9} = texttt{18}$ which gives us $texttt{1888}$
- Since we're still in the first left-right traversal and there were still two $texttt{8}$s we need to first replace these
- So we get $texttt{1816}$
- Something changed, so we need to do another iteration
- But there are no such digits, so we stop
Therefore the $9988^text{th}$ number in that sequence is $1816$.
Challenge
The first 200 terms are:
0,1,2,3,4,5,6,7,8,9,10,2,12,13,14,15,16,17,18,19,20,21,4,23,24,25,26,27,28,29,30,31,32,6,34,35,36,37,38,39,40,41,42,43,8,45,46,47,48,49,50,51,52,53,54,10,56,57,58,59,60,61,62,63,64,65,12,67,68,69,70,71,72,73,74,75,76,14,78,79,80,81,82,83,84,85,86,87,16,89,90,91,92,93,94,95,96,97,98,18,10,101,102,103,104,105,106,107,108,109,20,21,4,23,24,25,26,27,28,29,120,121,14,123,124,125,126,127,128,129,130,131,132,16,134,135,136,137,138,139,140,141,142,143,18,145,146,147,148,149,150,151,152,153,154,20,156,157,158,159,160,161,162,163,164,165,4,167,168,169,170,171,172,173,174,175,176,24,178,179,180,181,182,183,184,185,186,187,26,189,190,191,192,193,194,195,196,197,198,28
Your task is to generate that sequence, either
- given $n$, return the $n^text{th}$ number in that sequence,
- given $n$, return the first $n$ numbers in that sequence
- or generate the sequence indefinitely.
You may choose your submission to use either $0$- or $1$-indexing, but please specify which.
Test cases
You may use the above given terms, however here are some larger ones:
222 -> 42
1633 -> 4
4488 -> 816
15519 -> 2019
19988 -> 2816
99999 -> 18189
119988 -> 21816
100001 -> 101
999999 -> 181818
code-golf sequence integer
add a comment |
Let's define the a function on natural numbers $n$, written as base 10 digits $d_k; d_{k-1}; dotsc; d_1; d_0$, as follows:
As long as there are equal adjacent digits $d_i;d_{i-1}$, replace them by their sum $d_i+d_{i-1}$ from left to right. If there were any such digits, repeat the same procedure.
In other words, in each iteration we greedily take all pairs of equal adjacent digits and replace them by their sum at the same time (using the left-most pair if they overlap).
Example
Let's take $texttt{9988}$ for example:
- The first adjacent digits which are equal are the two $texttt{9}$
- So we replace them by $texttt{9 + 9} = texttt{18}$ which gives us $texttt{1888}$
- Since we're still in the first left-right traversal and there were still two $texttt{8}$s we need to first replace these
- So we get $texttt{1816}$
- Something changed, so we need to do another iteration
- But there are no such digits, so we stop
Therefore the $9988^text{th}$ number in that sequence is $1816$.
Challenge
The first 200 terms are:
0,1,2,3,4,5,6,7,8,9,10,2,12,13,14,15,16,17,18,19,20,21,4,23,24,25,26,27,28,29,30,31,32,6,34,35,36,37,38,39,40,41,42,43,8,45,46,47,48,49,50,51,52,53,54,10,56,57,58,59,60,61,62,63,64,65,12,67,68,69,70,71,72,73,74,75,76,14,78,79,80,81,82,83,84,85,86,87,16,89,90,91,92,93,94,95,96,97,98,18,10,101,102,103,104,105,106,107,108,109,20,21,4,23,24,25,26,27,28,29,120,121,14,123,124,125,126,127,128,129,130,131,132,16,134,135,136,137,138,139,140,141,142,143,18,145,146,147,148,149,150,151,152,153,154,20,156,157,158,159,160,161,162,163,164,165,4,167,168,169,170,171,172,173,174,175,176,24,178,179,180,181,182,183,184,185,186,187,26,189,190,191,192,193,194,195,196,197,198,28
Your task is to generate that sequence, either
- given $n$, return the $n^text{th}$ number in that sequence,
- given $n$, return the first $n$ numbers in that sequence
- or generate the sequence indefinitely.
You may choose your submission to use either $0$- or $1$-indexing, but please specify which.
Test cases
You may use the above given terms, however here are some larger ones:
222 -> 42
1633 -> 4
4488 -> 816
15519 -> 2019
19988 -> 2816
99999 -> 18189
119988 -> 21816
100001 -> 101
999999 -> 181818
code-golf sequence integer
Let's define the a function on natural numbers $n$, written as base 10 digits $d_k; d_{k-1}; dotsc; d_1; d_0$, as follows:
As long as there are equal adjacent digits $d_i;d_{i-1}$, replace them by their sum $d_i+d_{i-1}$ from left to right. If there were any such digits, repeat the same procedure.
In other words, in each iteration we greedily take all pairs of equal adjacent digits and replace them by their sum at the same time (using the left-most pair if they overlap).
Example
Let's take $texttt{9988}$ for example:
- The first adjacent digits which are equal are the two $texttt{9}$
- So we replace them by $texttt{9 + 9} = texttt{18}$ which gives us $texttt{1888}$
- Since we're still in the first left-right traversal and there were still two $texttt{8}$s we need to first replace these
- So we get $texttt{1816}$
- Something changed, so we need to do another iteration
- But there are no such digits, so we stop
Therefore the $9988^text{th}$ number in that sequence is $1816$.
Challenge
The first 200 terms are:
0,1,2,3,4,5,6,7,8,9,10,2,12,13,14,15,16,17,18,19,20,21,4,23,24,25,26,27,28,29,30,31,32,6,34,35,36,37,38,39,40,41,42,43,8,45,46,47,48,49,50,51,52,53,54,10,56,57,58,59,60,61,62,63,64,65,12,67,68,69,70,71,72,73,74,75,76,14,78,79,80,81,82,83,84,85,86,87,16,89,90,91,92,93,94,95,96,97,98,18,10,101,102,103,104,105,106,107,108,109,20,21,4,23,24,25,26,27,28,29,120,121,14,123,124,125,126,127,128,129,130,131,132,16,134,135,136,137,138,139,140,141,142,143,18,145,146,147,148,149,150,151,152,153,154,20,156,157,158,159,160,161,162,163,164,165,4,167,168,169,170,171,172,173,174,175,176,24,178,179,180,181,182,183,184,185,186,187,26,189,190,191,192,193,194,195,196,197,198,28
Your task is to generate that sequence, either
- given $n$, return the $n^text{th}$ number in that sequence,
- given $n$, return the first $n$ numbers in that sequence
- or generate the sequence indefinitely.
You may choose your submission to use either $0$- or $1$-indexing, but please specify which.
Test cases
You may use the above given terms, however here are some larger ones:
222 -> 42
1633 -> 4
4488 -> 816
15519 -> 2019
19988 -> 2816
99999 -> 18189
119988 -> 21816
100001 -> 101
999999 -> 181818
code-golf sequence integer
code-golf sequence integer
edited 40 mins ago
asked 1 hour ago


BMO
11.4k22185
11.4k22185
add a comment |
add a comment |
6 Answers
6
active
oldest
votes
Jelly, 11 bytes
DŒg+2/€FVµ¡
This is an unnecessarily slow, full program.
Try it online!
Alternate version, 12 bytes
DŒg+2/€FVµƬṪ
One byte longer, but much faster. Works as a program or a function.
Try it online!
How it works
DŒg+2/€FVµƬṪ Main link. Argument: n (integer)
µ Combine the previous links into a chain. Begin a new one.
D Decimal; yield n's digit array in base 10.
Œg Group adjacent, identical digits into subarrays.
+2/€ Map non-overlapping, pairwise sum over the subarrays.
If there is an odd number of digits in a subarray, the
last digit will remain untouched.
F Flatten; dump all sums and digits into a single array.
V Eval; turn the result into an integer.
Ƭ Execute the chain 'til the results are no longer unique.
Return all unique results.
Ṫ Tail; extract the last result.
The 11-byte version does the same, except it calls the link n times for input n, instead of calling it until a fixed point is reached.
add a comment |
JavaScript, 48 bytes
Input and output as strings. Returns the nth
term of the sequence.
f=s=>s==(s=s.replace(/(.)1/g,x=>x[0]*2))?s:f(s)
Try it online
add a comment |
Python 2, 97 96 93 bytes
def f(n):r=re.sub(r'(.)1',lambda m:`int(m.group(1))*2`,n);return r!=n and f(r)or r
import re
Try it online!
Non regex version:
Python 2, 133 130 122 112 98 bytes
def f(n):
r='';s=n
while s:a=1+(s[0]==s[1:2]);r+=`int(s[0])*a`;s=s[a:]
return r!=n and f(r)or r
Try it online!
add a comment |
Japt v2.0a0 -h
, 15 bytes
Input and output as strings. Returns the nth
term of the sequence.
_r/(.)1/ÏÑÃ}hN
Try it
This should work for 10 bytes but there seems to be a bug in Japt's recursive replacement method.
e/(.)1/ÏÑ
add a comment |
C# (.NET Core), 231 bytes
Must have:Using System.Linq;
which takes it from 214 -> 231 bytes.
Utilizes a string input/output.
p=>{bool f=true;while(f){int t=p.Select(n=>(n-'0')).ToArray();p="";f=false;var h=t.Length;for(var j=0;j<h;j++){if(j<h-1&&t[j]==t[j+1]){p+=t[j]+t[j+1];j++;f=true;continue;}p+=t[j];}}System.Console.Write(p+"n");}
Try it online!
The trailing new-line is not needed, you can also return the value instead of printing it which saves you bytes and1>0
is shorter thantrue
(same with `false). This brings you down to 190 bytes.
– BMO
5 mins ago
add a comment |
05AB1E, 9 bytes
Δγε2ôSO}J
Try it online!
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
return StackExchange.using("mathjaxEditing", function () {
StackExchange.MarkdownEditor.creationCallbacks.add(function (editor, postfix) {
StackExchange.mathjaxEditing.prepareWmdForMathJax(editor, postfix, [["\$", "\$"]]);
});
});
}, "mathjax-editing");
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "200"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodegolf.stackexchange.com%2fquestions%2f178256%2fcollapsing-numbers%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
6 Answers
6
active
oldest
votes
6 Answers
6
active
oldest
votes
active
oldest
votes
active
oldest
votes
Jelly, 11 bytes
DŒg+2/€FVµ¡
This is an unnecessarily slow, full program.
Try it online!
Alternate version, 12 bytes
DŒg+2/€FVµƬṪ
One byte longer, but much faster. Works as a program or a function.
Try it online!
How it works
DŒg+2/€FVµƬṪ Main link. Argument: n (integer)
µ Combine the previous links into a chain. Begin a new one.
D Decimal; yield n's digit array in base 10.
Œg Group adjacent, identical digits into subarrays.
+2/€ Map non-overlapping, pairwise sum over the subarrays.
If there is an odd number of digits in a subarray, the
last digit will remain untouched.
F Flatten; dump all sums and digits into a single array.
V Eval; turn the result into an integer.
Ƭ Execute the chain 'til the results are no longer unique.
Return all unique results.
Ṫ Tail; extract the last result.
The 11-byte version does the same, except it calls the link n times for input n, instead of calling it until a fixed point is reached.
add a comment |
Jelly, 11 bytes
DŒg+2/€FVµ¡
This is an unnecessarily slow, full program.
Try it online!
Alternate version, 12 bytes
DŒg+2/€FVµƬṪ
One byte longer, but much faster. Works as a program or a function.
Try it online!
How it works
DŒg+2/€FVµƬṪ Main link. Argument: n (integer)
µ Combine the previous links into a chain. Begin a new one.
D Decimal; yield n's digit array in base 10.
Œg Group adjacent, identical digits into subarrays.
+2/€ Map non-overlapping, pairwise sum over the subarrays.
If there is an odd number of digits in a subarray, the
last digit will remain untouched.
F Flatten; dump all sums and digits into a single array.
V Eval; turn the result into an integer.
Ƭ Execute the chain 'til the results are no longer unique.
Return all unique results.
Ṫ Tail; extract the last result.
The 11-byte version does the same, except it calls the link n times for input n, instead of calling it until a fixed point is reached.
add a comment |
Jelly, 11 bytes
DŒg+2/€FVµ¡
This is an unnecessarily slow, full program.
Try it online!
Alternate version, 12 bytes
DŒg+2/€FVµƬṪ
One byte longer, but much faster. Works as a program or a function.
Try it online!
How it works
DŒg+2/€FVµƬṪ Main link. Argument: n (integer)
µ Combine the previous links into a chain. Begin a new one.
D Decimal; yield n's digit array in base 10.
Œg Group adjacent, identical digits into subarrays.
+2/€ Map non-overlapping, pairwise sum over the subarrays.
If there is an odd number of digits in a subarray, the
last digit will remain untouched.
F Flatten; dump all sums and digits into a single array.
V Eval; turn the result into an integer.
Ƭ Execute the chain 'til the results are no longer unique.
Return all unique results.
Ṫ Tail; extract the last result.
The 11-byte version does the same, except it calls the link n times for input n, instead of calling it until a fixed point is reached.
Jelly, 11 bytes
DŒg+2/€FVµ¡
This is an unnecessarily slow, full program.
Try it online!
Alternate version, 12 bytes
DŒg+2/€FVµƬṪ
One byte longer, but much faster. Works as a program or a function.
Try it online!
How it works
DŒg+2/€FVµƬṪ Main link. Argument: n (integer)
µ Combine the previous links into a chain. Begin a new one.
D Decimal; yield n's digit array in base 10.
Œg Group adjacent, identical digits into subarrays.
+2/€ Map non-overlapping, pairwise sum over the subarrays.
If there is an odd number of digits in a subarray, the
last digit will remain untouched.
F Flatten; dump all sums and digits into a single array.
V Eval; turn the result into an integer.
Ƭ Execute the chain 'til the results are no longer unique.
Return all unique results.
Ṫ Tail; extract the last result.
The 11-byte version does the same, except it calls the link n times for input n, instead of calling it until a fixed point is reached.
edited 39 mins ago
answered 1 hour ago


Dennis♦
186k32296735
186k32296735
add a comment |
add a comment |
JavaScript, 48 bytes
Input and output as strings. Returns the nth
term of the sequence.
f=s=>s==(s=s.replace(/(.)1/g,x=>x[0]*2))?s:f(s)
Try it online
add a comment |
JavaScript, 48 bytes
Input and output as strings. Returns the nth
term of the sequence.
f=s=>s==(s=s.replace(/(.)1/g,x=>x[0]*2))?s:f(s)
Try it online
add a comment |
JavaScript, 48 bytes
Input and output as strings. Returns the nth
term of the sequence.
f=s=>s==(s=s.replace(/(.)1/g,x=>x[0]*2))?s:f(s)
Try it online
JavaScript, 48 bytes
Input and output as strings. Returns the nth
term of the sequence.
f=s=>s==(s=s.replace(/(.)1/g,x=>x[0]*2))?s:f(s)
Try it online
edited 55 mins ago
answered 1 hour ago


Shaggy
18.9k21666
18.9k21666
add a comment |
add a comment |
Python 2, 97 96 93 bytes
def f(n):r=re.sub(r'(.)1',lambda m:`int(m.group(1))*2`,n);return r!=n and f(r)or r
import re
Try it online!
Non regex version:
Python 2, 133 130 122 112 98 bytes
def f(n):
r='';s=n
while s:a=1+(s[0]==s[1:2]);r+=`int(s[0])*a`;s=s[a:]
return r!=n and f(r)or r
Try it online!
add a comment |
Python 2, 97 96 93 bytes
def f(n):r=re.sub(r'(.)1',lambda m:`int(m.group(1))*2`,n);return r!=n and f(r)or r
import re
Try it online!
Non regex version:
Python 2, 133 130 122 112 98 bytes
def f(n):
r='';s=n
while s:a=1+(s[0]==s[1:2]);r+=`int(s[0])*a`;s=s[a:]
return r!=n and f(r)or r
Try it online!
add a comment |
Python 2, 97 96 93 bytes
def f(n):r=re.sub(r'(.)1',lambda m:`int(m.group(1))*2`,n);return r!=n and f(r)or r
import re
Try it online!
Non regex version:
Python 2, 133 130 122 112 98 bytes
def f(n):
r='';s=n
while s:a=1+(s[0]==s[1:2]);r+=`int(s[0])*a`;s=s[a:]
return r!=n and f(r)or r
Try it online!
Python 2, 97 96 93 bytes
def f(n):r=re.sub(r'(.)1',lambda m:`int(m.group(1))*2`,n);return r!=n and f(r)or r
import re
Try it online!
Non regex version:
Python 2, 133 130 122 112 98 bytes
def f(n):
r='';s=n
while s:a=1+(s[0]==s[1:2]);r+=`int(s[0])*a`;s=s[a:]
return r!=n and f(r)or r
Try it online!
edited 53 mins ago
answered 1 hour ago


TFeld
14.2k21240
14.2k21240
add a comment |
add a comment |
Japt v2.0a0 -h
, 15 bytes
Input and output as strings. Returns the nth
term of the sequence.
_r/(.)1/ÏÑÃ}hN
Try it
This should work for 10 bytes but there seems to be a bug in Japt's recursive replacement method.
e/(.)1/ÏÑ
add a comment |
Japt v2.0a0 -h
, 15 bytes
Input and output as strings. Returns the nth
term of the sequence.
_r/(.)1/ÏÑÃ}hN
Try it
This should work for 10 bytes but there seems to be a bug in Japt's recursive replacement method.
e/(.)1/ÏÑ
add a comment |
Japt v2.0a0 -h
, 15 bytes
Input and output as strings. Returns the nth
term of the sequence.
_r/(.)1/ÏÑÃ}hN
Try it
This should work for 10 bytes but there seems to be a bug in Japt's recursive replacement method.
e/(.)1/ÏÑ
Japt v2.0a0 -h
, 15 bytes
Input and output as strings. Returns the nth
term of the sequence.
_r/(.)1/ÏÑÃ}hN
Try it
This should work for 10 bytes but there seems to be a bug in Japt's recursive replacement method.
e/(.)1/ÏÑ
answered 36 mins ago


Shaggy
18.9k21666
18.9k21666
add a comment |
add a comment |
C# (.NET Core), 231 bytes
Must have:Using System.Linq;
which takes it from 214 -> 231 bytes.
Utilizes a string input/output.
p=>{bool f=true;while(f){int t=p.Select(n=>(n-'0')).ToArray();p="";f=false;var h=t.Length;for(var j=0;j<h;j++){if(j<h-1&&t[j]==t[j+1]){p+=t[j]+t[j+1];j++;f=true;continue;}p+=t[j];}}System.Console.Write(p+"n");}
Try it online!
The trailing new-line is not needed, you can also return the value instead of printing it which saves you bytes and1>0
is shorter thantrue
(same with `false). This brings you down to 190 bytes.
– BMO
5 mins ago
add a comment |
C# (.NET Core), 231 bytes
Must have:Using System.Linq;
which takes it from 214 -> 231 bytes.
Utilizes a string input/output.
p=>{bool f=true;while(f){int t=p.Select(n=>(n-'0')).ToArray();p="";f=false;var h=t.Length;for(var j=0;j<h;j++){if(j<h-1&&t[j]==t[j+1]){p+=t[j]+t[j+1];j++;f=true;continue;}p+=t[j];}}System.Console.Write(p+"n");}
Try it online!
The trailing new-line is not needed, you can also return the value instead of printing it which saves you bytes and1>0
is shorter thantrue
(same with `false). This brings you down to 190 bytes.
– BMO
5 mins ago
add a comment |
C# (.NET Core), 231 bytes
Must have:Using System.Linq;
which takes it from 214 -> 231 bytes.
Utilizes a string input/output.
p=>{bool f=true;while(f){int t=p.Select(n=>(n-'0')).ToArray();p="";f=false;var h=t.Length;for(var j=0;j<h;j++){if(j<h-1&&t[j]==t[j+1]){p+=t[j]+t[j+1];j++;f=true;continue;}p+=t[j];}}System.Console.Write(p+"n");}
Try it online!
C# (.NET Core), 231 bytes
Must have:Using System.Linq;
which takes it from 214 -> 231 bytes.
Utilizes a string input/output.
p=>{bool f=true;while(f){int t=p.Select(n=>(n-'0')).ToArray();p="";f=false;var h=t.Length;for(var j=0;j<h;j++){if(j<h-1&&t[j]==t[j+1]){p+=t[j]+t[j+1];j++;f=true;continue;}p+=t[j];}}System.Console.Write(p+"n");}
Try it online!
answered 22 mins ago
Destroigo
312
312
The trailing new-line is not needed, you can also return the value instead of printing it which saves you bytes and1>0
is shorter thantrue
(same with `false). This brings you down to 190 bytes.
– BMO
5 mins ago
add a comment |
The trailing new-line is not needed, you can also return the value instead of printing it which saves you bytes and1>0
is shorter thantrue
(same with `false). This brings you down to 190 bytes.
– BMO
5 mins ago
The trailing new-line is not needed, you can also return the value instead of printing it which saves you bytes and
1>0
is shorter than true
(same with `false). This brings you down to 190 bytes.– BMO
5 mins ago
The trailing new-line is not needed, you can also return the value instead of printing it which saves you bytes and
1>0
is shorter than true
(same with `false). This brings you down to 190 bytes.– BMO
5 mins ago
add a comment |
05AB1E, 9 bytes
Δγε2ôSO}J
Try it online!
add a comment |
05AB1E, 9 bytes
Δγε2ôSO}J
Try it online!
add a comment |
05AB1E, 9 bytes
Δγε2ôSO}J
Try it online!
05AB1E, 9 bytes
Δγε2ôSO}J
Try it online!
answered 10 mins ago


Mr. Xcoder
31.6k759198
31.6k759198
add a comment |
add a comment |
If this is an answer to a challenge…
…Be sure to follow the challenge specification. However, please refrain from exploiting obvious loopholes. Answers abusing any of the standard loopholes are considered invalid. If you think a specification is unclear or underspecified, comment on the question instead.
…Try to optimize your score. For instance, answers to code-golf challenges should attempt to be as short as possible. You can always include a readable version of the code in addition to the competitive one.
Explanations of your answer make it more interesting to read and are very much encouraged.…Include a short header which indicates the language(s) of your code and its score, as defined by the challenge.
More generally…
…Please make sure to answer the question and provide sufficient detail.
…Avoid asking for help, clarification or responding to other answers (use comments instead).
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodegolf.stackexchange.com%2fquestions%2f178256%2fcollapsing-numbers%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
3WoPmWJ6 AuNDW